Click here to see the problem details on LeetCode.
Reverse a string is a pretty common type of problem. We all know how to reverse a string. But in this problem, we are not allowed to reverse the entire string. We have to reverse only vowels. We all know about vowels.
Let’s see a visual example of this problem.
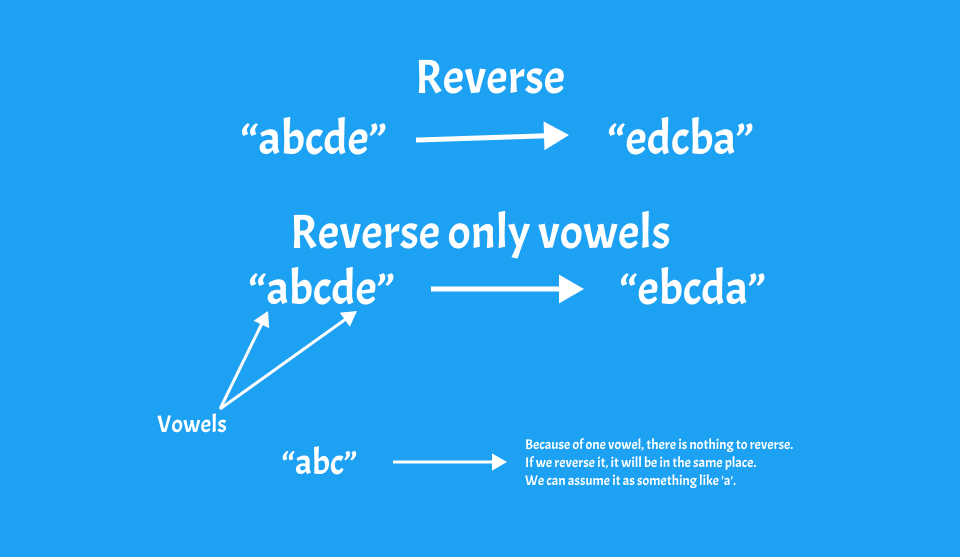
There are many ways to solve this problem. One of them is by using the stack data structure. So how can we do that?
First of all, we will store all vowels in a stack. After that, we will check each character of the given string. Each time we will check if it’s a vowel or not. If it’s not a vowel, then will store it into the final result. If it’s a vowel, we will store the last added character from the stack at this position instead of storing it. Because we all know that stack follows the rule Last In First Out. This way, we get the vowels from the end of the string to the beginning.
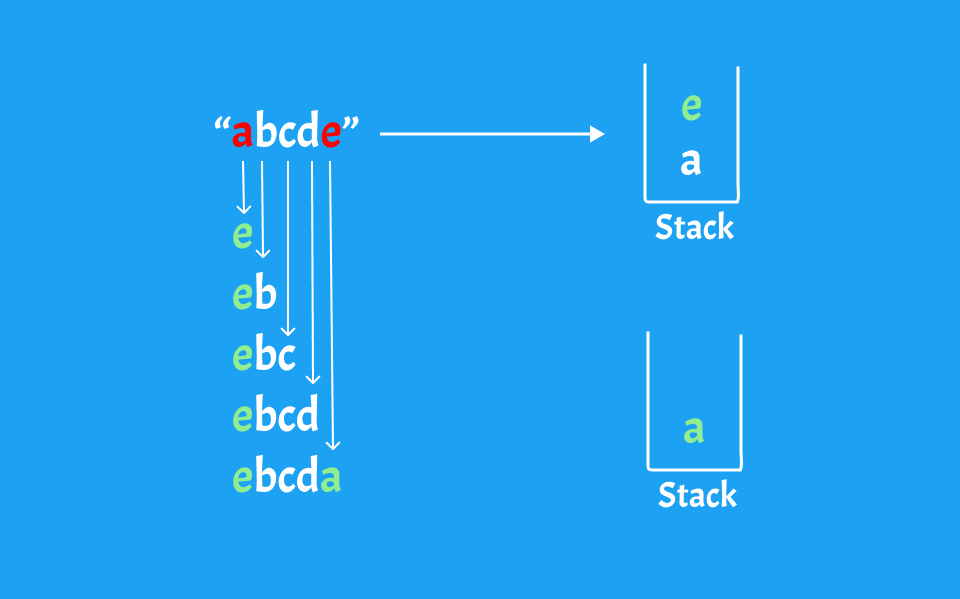
Let’s see the solution using code.
class Solution: def reverseVowels(self, s: str) -> str: stack = [] vowels = 'aeiouAEIOU' for let in s: if let in vowels: stack.append(let) result = '' for let in s: if let in vowels: result += stack.pop() else: result += let return result
Vowels can be in both upper and lower case. I hope you got the basic idea of this problem’s solution.