We all know that ReactJS is one of the most popular JavaScript frameworks/libraries. On the other hand, react-icons is an icon package.
Basically, react-icons are collections of various types of icon libraries in one place. FontAwesome is one of them. We can use many kinds of icons installing this one package in our project. It helps a lot. Personally, I find it pretty handy.
In this blog, I am going to use this package in a React project. Nothing fancy. I already created an empty React project. I know you can do it too. And I am going to show this in the App.js file.
Before using this package, we have to install it in our project. For that, use the following command.
npm install react-icons // or npm i react-icons // i is the short form of install
You can do it using yarn too.
yarn add react-icons
Now we can use icons in our project. For that, we have to import it. Let’s see the way of import.
import { IconName } from "react-icons/short-form-of-your-preferred-icon-library";
Click here to know about Icon Name and Icon Library name and others.
Let’s see how to use the Home icon from FontAwesome.
App.js
import './App.css'; import { FaHome } from 'react-icons/fa'; function App() { return ( <div className="app"> <h2>React Icons</h2> <h4> Home Icon From FontAwesome: <FaHome /> </h4> </div> ); } export default App;
App.css
.app { width: 500px; margin: 50px auto; background-color: #ddd; padding: 70px; }
Output
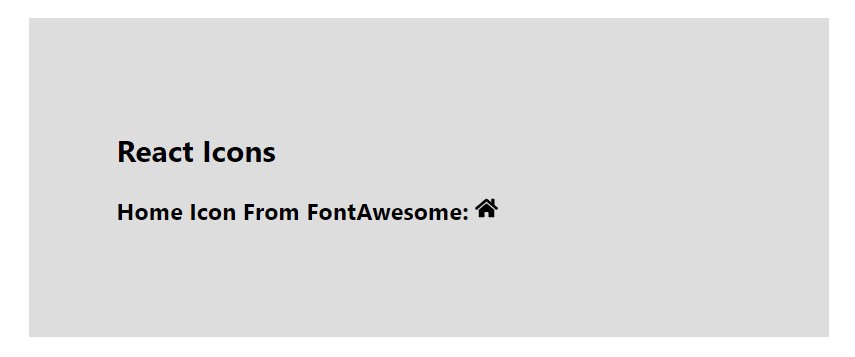
Let’s see an example of using another icon library (Flat Color Icons) in the same project.
App.js
import './App.css'; import { FaHome } from 'react-icons/fa'; import { FcHome } from 'react-icons/fc'; function App() { return ( <div className="app"> <h2>React Icons</h2> <h3> Home Icon From FontAwesome: <FaHome /> </h3> <h3> Home Icon From Flat Color Icons: <FcHome /> </h3> </div> ); } export default App;
Output
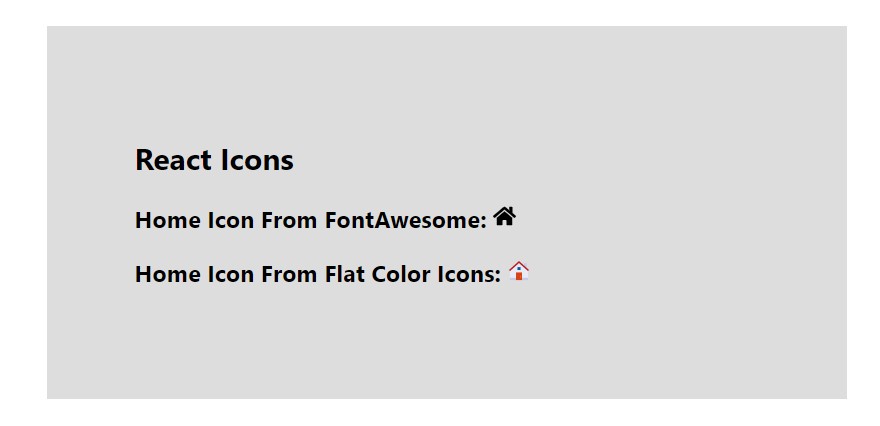
I hope you got an idea of how to use React Icons library/package. For more details and a list of icons, you can visit here.