Generating QR codes is super easy using Python. We will see how we can generate them using such a small amount of code in Python.
To do that, we first have to install a Python package. It is named
. To install it:qrcode
pip install qrcode
Then, just write the following code:
import qrcode def generate_qrcode(data, filename): img = qrcode.make(data) img.save(filename) print(f'QR code saved as {filename}') generate_qrcode('Generating QR codes is super easy using Python', 'result.png')
The above code is pretty straightforward. We have defined a function to generate a QR code, which takes two parameters. The first one is the data we want to encode in the QR code. The second one is the name of the image file.
Inside the function, we did mainly two simple things:
- Encode the given data in a QR code. (
)qrcode.make(data)
- Save the QR code to the given filename. (
)img.save(filename)
As we only gave the filename, it will save the image to the same directory where the script is located. You can also provide a specific file location here.
Now save the script file, and then run the script using Python, such as:
python script-file-name.py
Look at my generated QR code for the data ‘Generating QR codes is super easy using Python’.
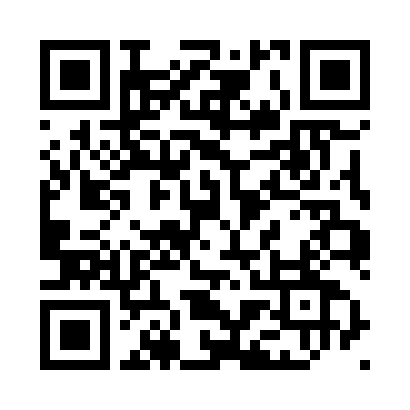
For further modifications, you can visit the package page on PyPI here.